Message Buffering Example
The following example walks through the scenario of adding packets through the API and how the session manages the internal buffers.
For this example, only the first two (internal) message buffers are shown. The type of session used for this example is a host session.
The following list provides a description of the session variables used in the example.
|
|
ThreasholdPtrs[]
|
Pointers to the end of each buffer, used to see if adding the packet would overrun the current buffer and if the
next one needs to be used (if one exists and is available). |
BufferPtrs[]
|
Pointers to the current position within each of the buffers. This is the location at which the next packet added
to the buffer will be placed. After a packet is added, this value is incremented to the address immediately after, where the
next packet is to be added. |
BasePtrs[]
|
Pointers to the beginning addresses of each of the buffers. This is the start of the space where the
CIGI_IG_CONTROL packet is added for host sessions and where the CIGI_START_OF_FRAME packet is added
for IG sessions. |
StartBufferIdx
|
The index of the buffer that marks the start of the outgoing message. This is the buffer that will be used as the
next outgoing message and is returned on the next call to CigiGetOutgoingMsgBuffer() . |
BufferIdx
|
The index of the "current" buffer which packets are added to. When the current buffer is full, the value
of this variable is updated to point to the next buffer for adding messages. The value of BuffIdxStartBuffIdx , if the starting buffer is full. |
|
Step 1: Start with an empty session. All buffers have been initialized and are empty.
StartBufferIdx: |
Points to the first buffer, which is also the buffer that will be the next CIGI message and is returned
on the next call to CigiGetOutgoingMsgBuffer(). |
|
BufferIdx: |
Points to the buffer also, since it is empty. This is the buffer to which packets will be added. |
|
BufferPtrs[0]: |
Points to the address where the next packet will be added, which is the beginning of the first buffer, immediately
after the space reserved for the CIGI_IG_CONTROL packet. |
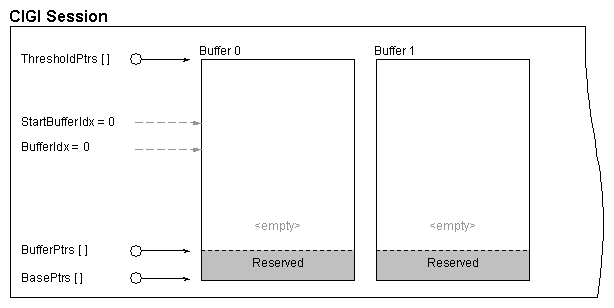 |
Figure 8 - Message Buffering Example Step 1 |
Step 2: Add an IG control and a entity control packet to the message. Note that the IG control packet was added to the beginning of the
message.
StartBufferIdx: |
Unchanged. |
|
BufferIdx: |
Unchanged, because the current buffer is not full. |
|
BufferPtrs[0]: |
Points to the address where the next packet will be added, immediately after the location of the
CIGI_ENTITY_CONTROL packet that was just added. |
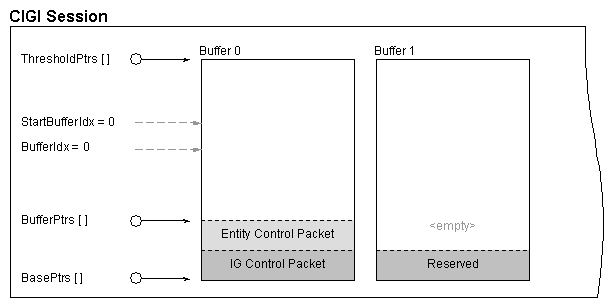 |
Figure 9 - Message Buffering Example Step 2 |
Step 3: Continue adding packets, filling up the first buffer. The buffer is not completely full, but there is no room remaining
at the end of the buffer to add another packet.
StartBufferIdx: |
Unchanged. |
|
BufferIdx: |
Unchanged. |
|
BufferPtrs[0]: |
Points to the address where the next packet would be added, but has not checked for space available to
add the next packet yet. |
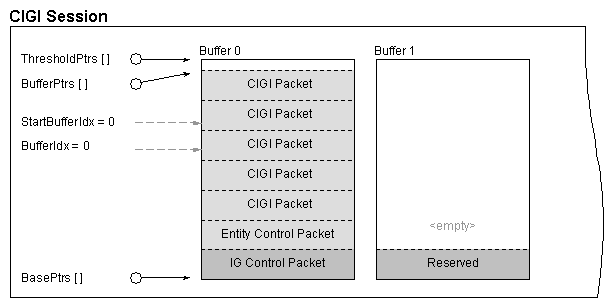 |
Figure 10 - Message Buffering Example Step 3 |
Step 4: Add an additional packet. Because there is not sufficient room in the current buffer, it will overflow into the next
buffer.
StartBufferIdx: |
Unchanged, still points to the message to be sent next. |
|
BufferIdx: |
Incremented to point to the next buffer, since the buffer that it was referring to is full. Packets added will be
added to the second buffer and will not go out when the next message is sent. |
|
BufferPtrs[1]: |
Points to the address where the next packet will be added, in the second (and current) message buffer. |
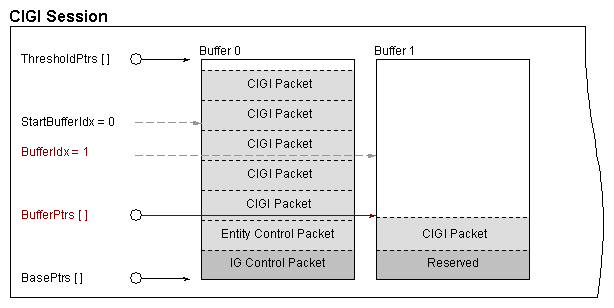 |
Figure 11 - Message Buffering Example Step 4 |
Step 5: End the current message with CigiEndMessage() and obtain the outgoing message buffer through the
CigiGetOutgoingMsgBuffer() function.. This is the entire contents of the first message buffer at index 0. Notice that the
message does not take up the entire contents of the message buffer, so only the portion of the buffer that the message uses needs to be sent.
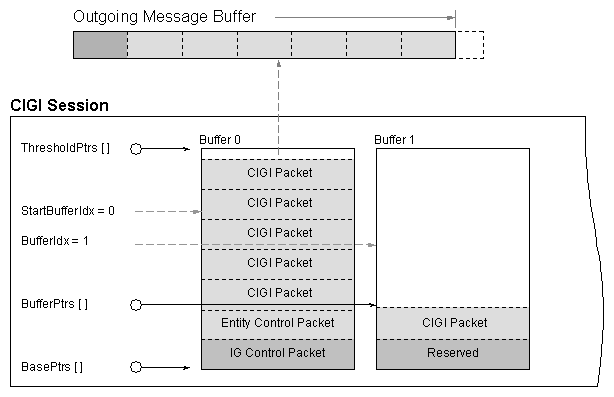 |
Figure 15 - Message Buffering Example Step 5 |
Step 6: Call the CigiStartMessage() function to begin a new message. Notice that the packet that was queued from an add
during the previous message is still in the message buffer.
StartBufferIdx: |
Advanced to refer to the next message buffer, since a new message was sent. This is the index of the buffer
that will be sent out as the next message. |
|
BufferIdx: |
Unchanged, since the position where packets are added to the message has not changed. |
|
BufferPtrs[1]: |
Unchanged. |
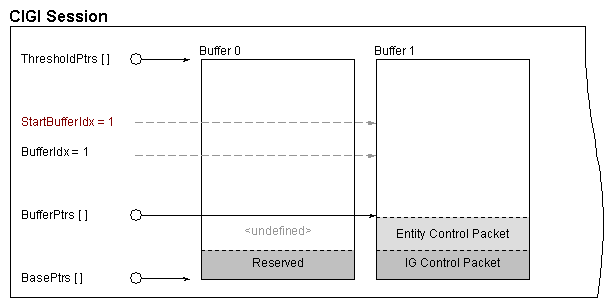 |
Figure 12 - Message Buffering Example Step 6 |
The processes described above repeats itself for the duration of the communication between the host and IG. The logic for advancing the message
buffer indexes is circular in nature, and when the end is reached it will loop back around to buffer 0, the first message buffer. The API will
not overwrite packets in the buffers if there is no room to queue an added packet. Instead, the function called to add the packet will return an
error code, such as CIGI_BUFFER_OVERRUN , and should be checked when adding the packet.
With this message buffering scheme, the API attempts to catch up on packets that do not fit into the outgoing message by queuing them in subsequent
messages. In this case, the size of the buffer should be incremented if possible, more buffers created, or less packets exchanged. Ideally, all
packets added during a given frame would go out on the same messages, but this may not be possible in some instances due to network limitations. If
packets are continually queued during ever frame, the buffer will be unable to catch up and eventually run out of room.
|
 |