Outgoing Messages
Packets are added to the outgoing CIGI message through the API. When the application is ready to send the outgoing message, it will obtain
the address of the packed message buffer from the API and is responsible for sending it. Note that the size of the buffer is fixed when the
session is created. The application however, does not need to send the entire buffer, but only the number of bytes that make up the CIGI
message. In other words, there may be extra room at the end of the outgoing buffer, and it does not need to be sent.
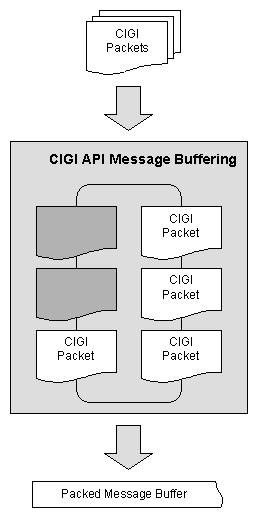 |
Figure 6 - Creating an Outgoing CIGI Message |
Packets are added to the outgoing message buffer one at a time. The API must be notified when starting a new outgoing messages or when ending
the current message so that it can send it. The application should start an outgoing message before adding packets to it, and the API assumes
that there is a current outgoing message started and that it has not ended yet. A message must be ended before it is sent.
All of the methods to add packets to the outgoing message have the same general form of: CigiAddPacketXXX() .
This can be seen in the prototype for adding an IG Control packet.
int CigiAddPacketIGCtrl(
const int sessionid,
const CIGI_IG_CONTROL* packet
);
|
 |
|
The sessionid argument indicates the ID received when the session is created using CigiCreateSession() , and the packet
argument is a pointer to the packet to add to the outgoing message. The data contained in the packet is copied into the outgoing message buffer
and the pointer value of the packet is not kept by the API after the function returns.
Starting and Ending the Outgoing Message
The API must be notified when outgoing messages start and end, and packets should only be added while there is a current message. Generally,
there will be exactly one outgoing message per start-of-frame. At a regular and interval, the IG should send a message each time it is starting
a frame to display. In response, the host should send a message back to the IG with the contents of the display for that frame. Thus, there
should be a one-to-one relationship between incoming and outgoing messages for both the host and the IG.
The prototypes for the CigiStartMessage() and CigiEndMessage() functions are as follows.
int CigiStartMessage(const int sessionid);
int CigiEndMessage(const int sessionid);
|
 |
|
Adding Packets to the Outgoing Message
The outgoing message for an IG should always contain a CIGI_START_OF_FRAME packet as the first packet, and will ultimately controls
the timing of the message delivery (at the start of each frame). In response, the host application is expected to respond immediately with a CIGI
message containing the data to display on the next frame. Host messages should always contain a CIGI_IG_CONTROL packet as the first
packet in the message.
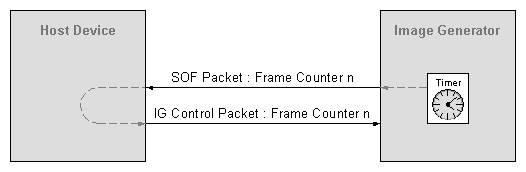 |
Figure 7 - First Packet for Host and IG Messages |
The CigiEndMessage() function for ending a message will return an error code if a CIGI_START_OF_FRAME packet has not
been added to an outgoing IG message, or if a CIGI_IG_CONTROL packet has not been added to an outgoing host message. These packets
do not necessarily have to be added first, since the API will place these packets at the start of the outgoing message. If multiple
CIGI_START_OF_FRAME or CIGI_IG_CONTROL packets are added, the previous message will be overwritten and only the packet
added last will appear at the beginning of the outgoing message.
Example 3 |
CigiStartMessage( nSessionId);
CigiAddPacketIGCtrl( nSessionId, pIGControl);
CigiAddPacketEntityCtrl( nSessionId, pEntityControl);
CigiAddPacketComponentCtrl( nSessionId, pComponentControl);
CigiEndMessage( nSessionId);
|
 |
|
Note: The packets added in between calls to CigiStartMessage() and CigiEndMessage() will not necessarily be
included in that message. If the total size of all packets added exceeds the size of the message buffer, packets will be added to back buffers,
if there is any room is available. The packets will be included in those messages instead. For a discussion on how packets are queued for
subsequent messages, see the message buffering example.
If all back buffers are full with queued packets, the function called to add the packet will return an error code.
Sending the Outgoing Message
The application is responsible for sending outgoing messages. Before sending the data, the CigiSyncFrameCounter() message should be
called. This will perform the following action.
- For IG sessions, it increments the internal frame counter and sets the frame counter in
the
CIGI_START_OF_FRAME packet to this value.
- For host sessions, it sets the frame counter in the
CIGI_IG_CONTROL packet
to the frame counter value received in incoming message buffer. note: For this method to function
properly for host sessions, the incoming message buffer must have been set with the last incoming message received so that the proper
frame counter is copied.
The prototype for the CigiSyncFrameCounter() function is as follows.
int CigiSyncFrameCounter(const int sessionid);
|
 |
|
The sessionid argument is the ID returned from CigiCreateSession() . When the message is ready to be sent, call the
CigiGetOutgoingMsgBuffer() function to obtain the outgoing message.
int CigiGetOutgoingMsgBuffer(
const int sessionid,
unsigned char **buffer,
int *size
);
|
 |
|
The sessionid argument is the ID returned from CigiCreateSession() . The buffer argument is the address of
the buffer pointer which will be set to the outgoing buffer. The size argument is size, in bytes, of the outgoing message. Only
the number of bytes equal to size needs to be sent. This is illustrated in the following example.
Example 4 : Sending the Outgoing Message |
int nSize = 0;
unsigned char * pBuffer = NULL;
CigiSyncFrameCounter( nSessionId);
CigiGetOutgoingMsgBuffer( nSessionId, &pBuffer, &nSize);
int nBytesSent = SendDataToIG( pBuffer, nSize);
...
|
 |
|
|
 |