Processing Incoming Data
There are two ways in which the API can examine the packets contained in an incoming CIGI message.
- Iteration functions.
- Callback functions.
Iteration Method for Processing Incoming Messages
To iterate through an incoming message, make sure to pass the incoming message buffer to the API through the
CigiSetIncomingMsgBuffer() function. Refer to the section on incoming messages for more information. The
prototypes for these two functions as follows.
int CigiGetFirstPacket(
const int sessionid,
unsigned char **packet
);
int CigiGetNextPacket(
const int sessionid,
unsigned char **packet
);
|
 |
|
The return value of this function is the CIGI opcode (packet ID) for the packet obtained. If the packet is not valid, or if all packets
in the message have been examined, CIGI_OPCODE_NONE will be returned, which is defined as 0 and is not a valid CIGI opcode. Each
time one of these functions is called, the return value should be checked for CIGI_OPCODE_NONE , to see if there are any packets
left to examine in the incoming message.
In each of these functions, the sessionid argument is the ID returned from CigiCreateSession() .
The packet argument will be set to point to the starting address of the packet received. This value will need to be type-casted to a
pointer of the correct CIGI packet type, depending on the return value. This pointer is also used to keep a position marker into the
incoming message buffer, and should not be modified. It is used to find the next packet in the message. The same packet variable should be
used for all calls to CigiGetFirstPacket() and CigiGetNextPacket() .
The CigiGetFirstPacket() function always returns the first packet in the message. The CigiGetNextPacket() function
returns the packet following the one passed in as the packet parameter. In order to reset the iteration, call CigiGetFirstPacket() .
Example 5 : Iterating through the Incoming Message |
unsigned char * pPacket = NULL;
int nOpcode = CigiGetFirstPacket( nSessionId, &pPacket);
while ( nOpcode != CIGI_OPCODE_NONE) {
switch ( nOpcode) {
case ( CIGI_SOF_OPCODE):
ProcessSOF( ( CIGI_START_OF_FRAME*)pPacket);
break ;
}
nOpcode = CigiGetNextPacket( nSessionId, &pPacket);
}
|
 |
|
As in the preceding example, an application will typically use a switch statement to evaluate the packet type, with
a case for each opcode the application wants to process.
Callback Method for Processing Incoming Messages
To use callback methods for examining the packets in the incoming message, the application will need to define the application-specific
functions that are invoked each time a packet of a certain type (opcode) is encountered.
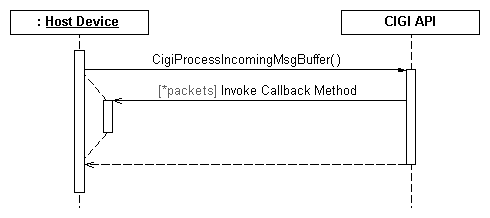 |
Figure 14 - Callback Sequence |
The type definition for the callback procedure and the prototype are listed below.
typedef long (* CIGICBPROC)(
const int sessionid,
void * packet
);
|
 |
|
Example 6 : Function Prototype for a Callback Function |
long ProcessIncomingIGControlPacket(
const int sessionid,
void * packet
);
|
 |
|
A call back function may be registered for each packet type that the application wants to process, indicated by the opcode. To do this,
call the CigiSetCallback() function.
int CigiSetCallback(
const int opcode,
const CIGICBPROC proc
);
|
 |
|
In addition, a default callback function may be set. The default callback function will be called each time an incoming packet
is encontered for which there is no callback function set for specifically (using the CigiSetCallback()
function).
void CigiSetDefaultCallback(
const CIGICBPROC proc
);
|
 |
|
To unset the default callback function for handling incoming packets, call this function with an argument
of NULL .
Note: One callback function can be mapped to more than one opcode. In this case the user will have to examine the packet to determine
the opcode. This can be done by casting the packet to an unsigned char type, since the first byte of each packet
contains the opcode. This is illustrated in the following example.
Example 7 : Determining the Opcode for a CIGI Packet |
int opcode = *( ( unsigned char *)packet);
|
 |
|
|